Hayden's Archive
[자바/Java] 도서관 사서 프로그램 작성하기 본문
- VO Class : Book, Magazine // Magazine은 Book으로부터 상속받는다
- Service Class : BookManager // BookManager는 Book과 Magazine을 관리한다. 그리고 여기에 Javadoc를 작성한다.
- Test Class : BookTest // 모든 출력은 BookTest에서 한다. BookManager의 요청을 처리할 수 있는 메뉴를 만들어본다.
- 위의 내용을 만족할 수 있는 Class Diagram을 작성하고 구현해본다.
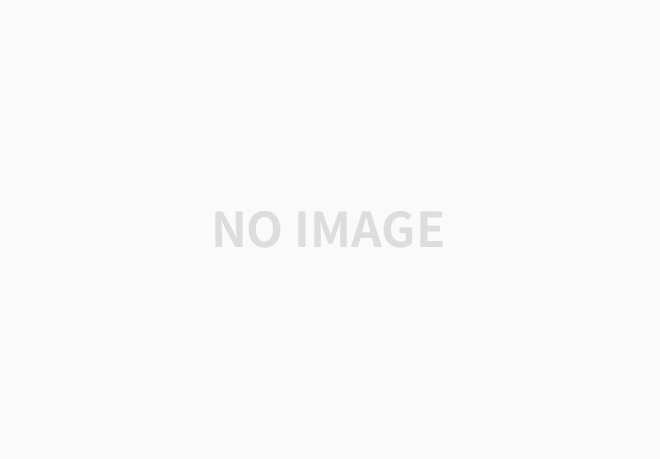
package com.encore.book;
public class Book {
private String isbn;
private String title;
private String author;
private String publisher;
private double price;
private String desc; // description. 간단한 설명
public Book() {};
public Book(String isbn, String title, String author, String publisher, double price, String desc) {
super();
this.isbn = isbn;
this.title = title;
this.author = author;
this.publisher = publisher;
this.price = price;
this.desc = desc;
}
public String getIsbn() {
return isbn;
}
public void setIsbn(String isbn) {
this.isbn = isbn;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
public String getDesc() {
return desc;
}
public void setDesc(String desc) {
this.desc = desc;
}
public String toString() {
return isbn +", "+title+", "+author+", "+publisher+", "+price+", "+desc;
}
}
package com.encore.magazine;
import com.encore.book.Book;
public class Magazine extends Book {
private int year;
private int month;
public Magazine(String isbn, String title, String author, String publisher, double price, String desc, int year, int month) {
super(isbn, title, author, publisher, price, desc);
this.year = year;
this.month = month;
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public String toString() {
return super.toString() + ", "+ year + ", "+ month;
}
}
package com.encore.bookmanager;
import com.encore.book.Book;
/**
*
* @author Hayden Jones
* @since JDK 1.8.5
* @version Start Version level 1.
*
*/
public class BookManager {
Book[] bList;
int numberOfBooks;
public BookManager(int numberOfBooks) {
bList = new Book[numberOfBooks];
}
/**
*
* @return 책 목록 배열로 도서관 소장책 권수를 반환함
*/
public int getNumberOfBooks() {
return numberOfBooks;
}
/**
* 새로운 도서를 배열 bList에 추가하고 책 권수를 1권 늘림
* 추가하려는 도서의 ISBN이 이미 보유하고 있는 도서의 ISBN과 같다면 추가하지 않음
* @param newBook 새로 추가할 Book 타입 책 객체
*/
public void insertBook(Book newBook) {
for(int i = 0; i < bList.length; i++) {
if(bList[i] == null) {
bList[i] = newBook;
numberOfBooks++;
break;
}
}
}
/**
* 배열 bList에 있는 요소 b가 null이라면 지나침
* @return 모든 책 객체를 요소로 가지는 배열 temp를 반환함
*/
public Book[] getAllBook() {
Book[] temp = new Book[numberOfBooks];
int i = 0;
for(Book b : bList) {
if(b == null) {
continue;
}
else if(b.equals(bList[i])) {
temp[i] = b;
}
i++;
}
return temp;
}
/**
*
* @param isbn ISBN을 입력함
* @return 해당하는 ISBN의 책 객체를 반환함
*/
public Book searchBook(String isbn) {
Book temp = null;
for(Book b : bList) {
if(b != null) {
if(b.getIsbn().equals(isbn)) {
temp = b;
}
}
}
return temp;
}
/**
*
* @param title 책 제목을 입력함
* @return 해당하는 제목의 책 객체들의 배열을 반환함
*/
public Book[] searchBookByTitle(String title) {
int count = 0;
int i = 0;
Book[] temp1 = new Book[numberOfBooks]; // 임시로 담을 배열 생성
for(Book b : bList) {
if(b != null) {
if(b.getTitle().equals(title)) {
temp1[i++] = b;
count++;
}
}
}
Book[] temp2 = new Book[count]; // 반환할 배열을 따로 만듦
for(int j = 0; j < count; j++) {
if(temp1[j] != null) {
temp2[j] = temp1[j];
}
}
return temp2;
}
/**
*
* @param publisher 출판사를 입력함
* @return 해당하는 출판사의 책 객체들의 배열을 반환함
*/
public Book[] searchBookByPublisher(String publisher) {
int count = 0;
int i = 0;
Book[] temp1 = new Book[numberOfBooks];
for(Book b : bList) {
if(b != null) {
if(b.getPublisher().equals(publisher)) {
temp1[i++] = b;
count++;
}
}
}
Book[] temp2 = new Book[count];
for(int j = 0; j < count; j++) {
if(temp1[j] != null) {
temp2[j] = temp1[j];
}
}
return temp2;
}
/**
* 타입을 double로 달리하여 Method Overloading 기법을 씀.
* @param price 책 가격을 입력함
* @return 해당하는 가격의 책 객체들의 배열을 반환함
*/
public Book[] searchBook(double price) {
int count = 0;
int i = 0;
Book[] temp1 = new Book[numberOfBooks];
for(Book b : bList) {
if(b != null) {
if(b.getPrice() == price) {
temp1[i++] = b;
count++;
}
}
}
Book[] temp2 = new Book[count];
for(int j = 0; j < count; j++) {
temp2[j] = temp1[j];
}
return temp2;
}
/**
*
* @return 모든 책의 가격을 합한 total을 반환함
*/
public double getSumPriceOfBooks() {
int total = 0;
for(Book b : bList) {
if(b != null) total += b.getPrice();
}
return total;
}
/**
*
* @return 모든 책의 가격을 합하고 개수만큼 나눠서 평균을 반환함
*/
public double getAveragePriceOfBooks() {
return getSumPriceOfBooks() / numberOfBooks;
}
}
package test;
import java.util.Scanner;
import com.encore.book.Book;
import com.encore.bookmanager.BookManager;
import com.encore.magazine.Magazine;
public class BookTest {
public static void main(String[] args) {// 소장책 DB
Book a = new Book("9788954620512", "데미안", "헤르만 헤세", "민음사", 8500.0, "불안한 젊음에 바치는 영혼의 자서전");
Book b = new Book("9788954620512", "데미안", "헤르만 헤세", "민음사", 8500.0, "불안한 젊음에 바치는 영혼의 자서전");
Book c = new Magazine("9788954620260", "어린이 과학동아", "편집부", "동아사이언스", 8500.0, "초등학생을 위해 한달에 두 번 발행하는 과학만화 잡지", 2020, 3);
Book d = new Book("9771975252008", "시간 가게", "이나영", "문학동네", 9900.0, "제 13회 문학동네 어린이 문학상 수상작");
Book e = new Magazine("9771228402006", "씨네21", "편집부", "씨네21", 3800.0, "대한민국의 영화 전문 잡지", 2020, 4);
Book[] bs = {a,b,c,d,e,};
printBooks(bs);
}
public static void printBooks(Book[] bs) {// 출력 메소드
BookManager service = new BookManager(100); // 도서 최대 보유권수는 100권으로 지정
// 메인 메소드의 배열에서 받아온 소장책 객체들을 service에 있는 배열에 추가함
for(int i = 0; i < bs.length; i++) {
service.insertBook(bs[i]);
}
boolean menu = true; // 메뉴 스위치
while(menu == true) { // 메뉴 스위치가 켜져있는 동안 무한반복
System.out.println("===============================");
System.out.println("S 전자 오픈 도서관 관리 페이지입니다.");
System.out.println("1. 도서관 전체 소장책 조회");
System.out.println("2. 도서관 소장책 추가");
System.out.println("3. 책 찾기");
System.out.println("4. 전체책 가격 합계 및 평균 조회");
System.out.println("===============================");
Scanner sc = new Scanner(System.in);
int choice = sc.nextInt(); // 메뉴 번호 입력
// 1. 도서관 전체 소장책 조회
if(choice == 1) {
System.out.println("전체 소장책 총 "+service.getNumberOfBooks()+"권");
for(Book b : service.getAllBook()) {
System.out.println(b);
}
}
// 2. 도서관 소장책 추가
else if(choice == 2) {
// service.insertBook(new Book("9788937460586", "싯다르타", "헤르만 헤세", "민음사", 6300.0, "헤세가 소설로 형상화한 부처의 생애"));
// service.insertBook(new Magazine("9771227130009", "뉴턴", "편집부", "아이뉴턴", 12000.0, "한중일에서 동시에 발행되고 있는 세계적인 과학 전문지", 2019, 11));
System.out.println("ISBN을 입력하세요.");
String isbn = sc.next();
System.out.println("책 제목을 입력하세요."); sc.nextLine();
String title = sc.nextLine();
System.out.println("작가를 입력하세요.");
String author = sc.nextLine();
System.out.println("출판사를 입력하세요.");
String publisher = sc.nextLine();
System.out.println("가격을 입력하세요.");
Double price = sc.nextDouble(); sc.nextLine();
System.out.println("간단한 설명을 입력하세요.");
String desc = sc.nextLine();
System.out.println("일반도서이면 true, 잡지이면 false를 입력하세요.");
Boolean bkOrMaga = sc.nextBoolean();
if(bkOrMaga.equals(true)) {
service.insertBook(new Book(isbn, title, author, publisher, price, desc));
}
else if(bkOrMaga.equals(false)) {
System.out.println("출간연도를 입력하세요.");
int year = sc.nextInt();
System.out.println("출간월을 입력하세요.");
int month = sc.nextInt();
service.insertBook(new Magazine(isbn, title, author, publisher, price, desc, year, month));
}
System.out.println("소장책이 추가되었습니다.");
}
// 3. 책 찾기
else if(choice == 3) {
System.out.println("===============================");
System.out.println("어떤 방법으로 책을 찾겠습니까?");
System.out.println("1. ISBN으로 책 찾기");
System.out.println("2. 책 제목으로 책 찾기");
System.out.println("3. 출판사로 책 찾기");
System.out.println("4. 가격으로 책 찾기");
System.out.println("5. 이전으로");
System.out.println("===============================");
sc.nextLine();
int search = sc.nextInt();
if(search == 1) {
System.out.println("ISBN을 입력하세요.");
String isbn = sc.next(); sc.nextLine();
if(service.searchBook(isbn) == null) System.out.println("일치하는 책이 없습니다.");
else System.out.println(service.searchBook(isbn));
}
else if(search == 2) {
System.out.println("책 제목을 입력하세요."); sc.nextLine();
String title = sc.nextLine();
int count = 0;
for(Book b : service.searchBookByTitle(title)) {
if(b != null) System.out.println(b);
else count++;
}
if(count == service.searchBookByTitle(title).length) {
System.out.println("일치하는 책이 없습니다.");
}
}
else if(search == 3) {
System.out.println("출판사를 입력하세요.");
String publisher = sc.next();
int count = 0;
for(Book b : service.searchBookByPublisher(publisher)) {
if(b != null) System.out.println(b);
else count++;
}
if(count == service.searchBookByPublisher(publisher).length) {
System.out.println("일치하는 책이 없습니다.");
}
}
else if(search == 4) {
System.out.println("가격을 입력하세요.");
double price = sc.nextDouble(); sc.nextLine();
int count = 0;
for(Book b : service.searchBook((double)price)) {
if(b != null) System.out.println(b);
else count++;
}
if(count == service.searchBook((double)price).length) {
System.out.println("일치하는 책이 없습니다.");
}
}
else if(search == 5) menu = true;
else System.out.println("잘못 입력하셨습니다.");
}
// 4. 전체책 가격 합계 및 평균 조회
else if(choice == 4) {
System.out.println("전체책 가격 합계 : " + service.getSumPriceOfBooks());
System.out.println("전체책 가격 평균 : " + service.getAveragePriceOfBooks());
}
// 잘못 입력했을 경우
else {
System.out.println("잘못 입력하셨습니다.");
}
}
}
}